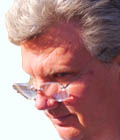 |
|
Conditional Statements
Linux Tips by Burleson Consulting |
An important aspect of
programming is being able to determine if a condition is true or false and then
perform specific actions depending upon the outcome of the condition test. This
can be especially useful when used with the exit status of the previous command.
Shell scripting supplies several ways of testing
conditions and then performing specific actions. Some examples of condition
statements are the if condition, the case statement and the test or expression
statement. Conditions are also tested in the while loop and the until loop and
specific actions are repeated.
The IF condition
The simple form of the if statement consists of the if
condition, a then statement followed by commands to be executed when the if
condition is true, and the IF statement (if spelled backwards) which ends the if
statement. The condition usually consists of a simple comparison between two
values, such as A=B or number=5. The following is a simple if conditional
statement.
$ x=3
$ y=9
$ if [ $x -lt $y ]
> then
> echo " "
> echo " x=${x} which is less than y=${y}"
> echo " "
> fi
x=3 which is less than y=9
The simple if statement also provides an else clause
which allows the execution of commands when the condition is false. The
following is an example of a simple if-then-else statement.
$ x=3
$ y=2
$ if [ $x -lt $y ]
> then
> echo " "
> echo " ${x} is less than ${y}"
> echo " "
> else
> echo " "
> echo " ${x} is not less than ${y}"
> echo " "
> fi
3 is not less than 2
It is also possible to create compound conditional
statements by using one or more else if (elif) clauses. If the first condition
is false, then subsequent elif statements are checked. When an elif condition
is found to be true, the statements following the associated then statement are
executed.
If the first condition and subsequent elif conditions
are false, statements following the else statement, if present, are executed.
The following script shows an example of compound conditional if statements.
$ x=5
$ y=5
$ if [ $x -lt $y ]
> then
> echo " "
> echo " ${x} is less than ${y}"
> echo " "
> elif [ $x -eq $y ]
> then
> echo " "
> echo " ${x} is equal to ${y}"
> echo " "
> else
> echo " "
> echo " ${x} is greater than ${y}"
> echo " "
> fi
5 is equal to 5
Now we'll run the exact same script but with different
values for x and y so we can test for another result.
$ x=5
$ y=3
$
$ if [ $x -lt $y ]
> then
> echo " "
> echo " ${x} is less than ${y}"
> echo " "
> elif [ $x -eq $y ]
> then
> echo " "
> echo " ${x} is equal to ${y}"
> echo " "
> else
> echo " "
> echo " ${x} is greater than ${y}"
> echo " "
> fi
5 is greater than 3
In the example above, -lt was as an operator to check
for a ?less than? condition, and -eq was used to check for an ?equal?
condition. Table 8.3 below contains a list of operators available for use when
evaluating conditional statements:
Operator |
Meaning |
Mathematical Equivalent |
-eq |
equal to |
x == y |
-ge |
greater than or equal to |
x >= y |
-gt |
greater than |
x > y |
-le |
less than or equal to |
x <= y |
-lt |
less than |
x < y |
-ne |
not equal to |
x != y |
Table 8.3: Operators available for conditional
statements and their meanings
Next we will look at how we can use test to check for
even more conditions.
Using the test Command or [ expression ]
Either the test command or just an expression in square
brackets ( [ expression ]) can be used to evaluate whether an expression is true
(zero) or false (non-zero). As the following example illustrates, an expression
evaluation can be accomplished in a number of different ways.
$ x=3
$ y=7
$ test $x -lt $y && echo " Option 1 -- ${x} is less
than ${y} "
Option 1 -- 3 is less than 7
$ if test $x -lt $y
> then
> echo " Option 2 -- ${x} is less than ${y} "
> fi
Option 2 -- 3 is less than 7
$ if [ $x -lt $y ]
> then
> echo " Option 3 -- ${x} is less than ${y} "
> fi
Option 3 -- 3 is less than 7
The three conditional statements above all test for the
exact same condition. In the first one, && is used to execute the echo command
if, and only if the exit status of the test command is 0.
The information in Table 8.4 below extracted from the
Linux man page for test shows some of the other conditions we can test for. The
information summarizes the different types of tests that can be performed on
files:
Test |
Description |
-b |
file exists and is block special |
-c |
file exists and is character special |
-d |
file exists and is a directory |
-e |
file exists |
-f |
file exists and is a regular file |
-g |
file exists and is set-group-ID |
-G |
file exists and is owned by the
effective group ID |
-h |
file exists and is a symbolic link
(same as -L |
-k |
file exists and has its sticky bit
set |
-L |
file exists and is a symbolic link
(same as -h) |
-O |
file exists and is owned by the
effective user ID |
-p |
file exists and is a named pipe |
-r |
file exists and is readable |
-S |
file exists and is a socket |
-s |
file exists and has a size greater
than zero |
-t |
file descriptor FD (stdout by
default) is opened on a terminal |
-u |
file exists and its set-user-ID bit
is set |
-w |
file exists and is writable |
-x |
file exists and is executable |
-nt |
file1 is newer (modification date)
than file2 |
-ot |
file1 is older than file2 |
-ef |
file1 and file2 have the same device
and inode numbers |
Table 8.4: Linux tests and their descriptions
This type of test can be useful to check if your script
relies on a certain file being around or writable for things to work right. The
following are file test examples:
$ if [ -e ".bash_profile" ]
> then
> echo " The file exists "
> else
> echo " File not found "
> fi
The file exists
$ if [ -e ".bash_proxxx" ]
> then
> echo " The file exists "
> else
> echo " File not found "
> fi
File not found
There are even more things we can test for when it comes
to comparing strings. Table 8.5 contains string tests and their descriptions.
Test |
Description |
!= |
the strings are not equal |
= |
the strings are equal |
-l |
evaluates to the length of the string |
-n |
the length of string is nonzero |
-z |
the length of string is zero |
Table 8.5: String tests and descriptions
The following examples illustrate one use of the string
test.
$ if [ -n "" ]
> then
> echo " The string contains characters"
> else
> echo " The string is empty "
> fi
The string is empty
$ if [ -n "ABC 123" ]
> then
> echo " The string contains characters"
> else
> echo " The string is empty "
> fi
The string contains characters
Any of these simple test conditions can be evaluated
individually or combined with other conditions to evaluate complex expressions.
Table 8.6 contains the connectives for test and their descriptions.
Connective |
Description |
! |
expression is false |
-a |
both expression1 and expression2 are
true (logical and) |
-o |
either expression1 or expression2 is
true (logical or) |
Table 8.6: Connectives and their descriptions
Here is how we could use the or connective to check two
separate conditions:
$ a="abc"
$ b="abc"
$ if [ "$a" = "xyz" -o "$a" = "$b" ]
> then
> echo " One of the conditions is true "
> else
> echo " Neither condition is true "
> fi
One of the conditions is true
$ a="abc"
$ b="xyz"
$ if [ "$a" = "xyz" -o "$a" = "$b" ]
> then
> echo " One of the conditions is true "
> else
> echo " Neither condition is true "
> fi
Neither condition is true
Complex conditions can be used to evaluate several
factors and react to certain conditions on the system or in the shell script.
Next we'll see how these conditions can be used within loops to repeat an
action.
Loops
A loop provides a way to execute commands repeatedly
until a condition is satisfied. The bash shell provides several looping methods
but we'll focus on the for loop and the while loop.
for loop
The for loop executes the commands placed between the do
and done statements until all values in the value list passed to it have been
processed. Here is a simple example of the for loop:
$ animals="dog cat bird mouse"
$
$ for animal in $animals
> do
> echo $animal
> done
dog
cat
bird
mouse
As we can see
here, we did not have to tell the for loop how many times to repeat. It just
took the input and repeated it for the number of separate items in the variable
$animals. It is easy to see how we might use this to parse over a list of
filenames from the user.
This is an excerpt from "Easy
Linux Commands" by Linux guru Jon Emmons. You can purchase it for only
$19.95 (30%-off) at
this link.